· Programming concepts - 24 min read
Comparing AOT and JIT Compilers: Understanding the Differences and Making an Informed Choice
In the world of programming, choosing between Ahead-Of-Time (AOT) and Just-In-Time (JIT) compilers can be crucial. We explore the key differences, benefits, and performance of each compiler to help you make an informed decision for your programming project.
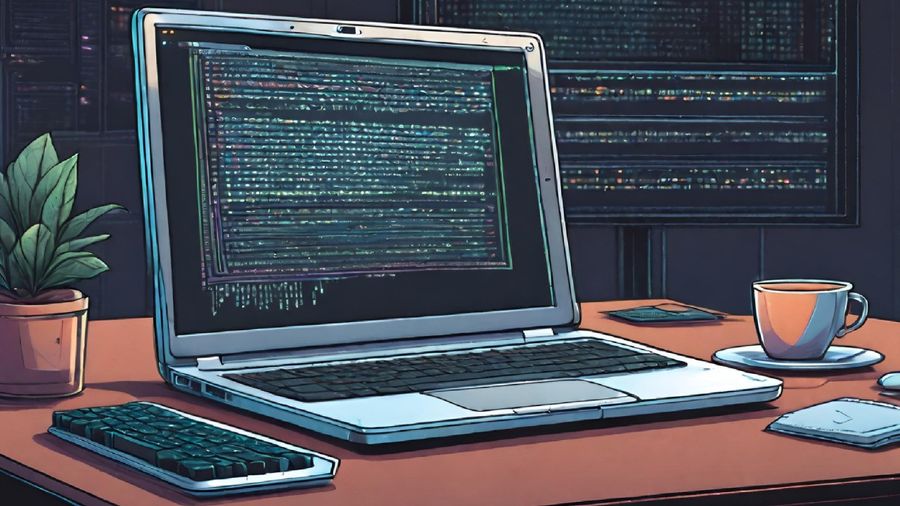
Introduction
IntroductionProgramming languages are the backbone of software development, enabling developers to write efficient and practical code. However, before a computer can execute code, it must first be transformed into a format that the machine can understand. This transformation is performed by compilers, which play a crucial role in translating human-readable code into machine-executable instructions. They optimize code and produce executable programs. In this post, we will examine the pros and cons of two well-known compiler types: Ahead-Of-Time (AOT) and Just-In-Time (JIT) compilers.
What is Just-In-Time (JIT) Compilation?
What is Just-In-Time (JIT) Compilation?Just-In-Time (JIT) compilation is a technique used by many modern programming languages and platforms to improve runtime performance. Instead of converting the entire source code into machine code before execution, JIT dynamically compiles and optimizes code during execution. This real-time compilation allows for optimizations based on the actual runtime behavior of the program. JIT compilers can produce highly dynamic code and enable advanced debugging features, making them particularly useful in interpreted languages such as JavaScript.
What is Ahead-Of-Time (AOT) Compilation?
What is Ahead-Of-Time (AOT) Compilation?Ahead-Of-Time (AOT) compilation is a process where the source code is converted into machine code before the program is executed. This often occurs during the build phase, preparing the machine code so that no further conversion is required at runtime. AOT typically improves application performance and reduces load time, allowing it to fully utilize the hardware infrastructure. This approach is particularly beneficial for applications that require fast startup times and consistent performance.
Compilation Process
Compilation ProcessLet’s explore how each of these techniques works.
How JIT Compilation Works
How JIT Compilation WorksJIT compilation occurs during the execution of the program. The process involves several stages:
Analysis
AnalysisThe portion of the code to be executed (instead of the entire source code) is analyzed for its structure and instructions.
Intermediate Representation (IR) Generation
Intermediate Representation (IR) GenerationThe analyzed code is converted into an intermediate representation (IR), such as bytecode or IL (Intermediate Language), which makes it easier to work with during the compilation process.
Optimization
OptimizationJIT compilers perform various optimizations to enhance performance. These optimizations may include removing redundant operations, simplifying expressions, combining functions, and identifying opportunities for parallel execution.
Dynamic Profiling
Dynamic ProfilingTo generate the most efficient machine code, JIT relies on dynamic profiling. It monitors the program during execution and collects data on the behavior of different code paths. This profiling helps JIT make better decisions about which optimizations to apply.
Code Generation
Code GenerationBased on the profiling data, the JIT compiler generates machine code tailored to the specific hardware architecture. This machine code is optimized for execution speed and may utilize hardware-specific features like vectorization or branch prediction.
Temporary Code Storage
Temporary Code StorageThe generated machine code is temporarily stored (cached) for reuse, improving execution speed by eliminating the need to recompile the same source code repeatedly.
Code Replacement
Code ReplacementIn some cases, JIT may replace previously compiled code with a newer version. This can happen when new profiling data suggests a better optimization strategy. Code replacement allows JIT to adapt dynamically to changing runtime conditions and improve performance.
Execution
ExecutionAfter the machine code is generated, it replaces the intermediate representation, and the program continues execution using the optimized machine code.
How AOT Compilation Works
How AOT Compilation WorksThe AOT compilation process involves several stages to convert high-level code into machine code that can be executed directly by the target hardware. Here’s an overview of the AOT compilation steps:
Static Analysis
Static AnalysisSource code, typically written in high-level languages like C, C++, or Go, undergoes static analysis to check for syntax errors, type checking, and other code analysis tasks.
Intermediate Representation Generation
Intermediate Representation GenerationAfter analysis, the source code is first converted into an intermediate representation (IR) to simplify the optimization process.
Optimization
OptimizationThe intermediate representation is optimized to enhance performance, reduce memory usage, and eliminate redundant operations. The specific optimizations depend on the compiler and target platform.
Machine Code Generation
Machine Code GenerationOnce optimized, the AOT compiler generates machine code specific to the target hardware architecture. This involves translating the IR into low-level instructions that can be executed directly by the processor.
Linking with Platform-Specific Dependencies
Linking with Platform-Specific DependenciesIf necessary, the compiled AOT code is linked with platform-specific libraries and dependencies. This ensures that the code interacts efficiently with the system’s infrastructure.
Binary Output
Binary OutputThe final output of the AOT compilation process is an executable binary file containing machine code specific to the target platform, ready for direct execution.
Installation and Deployment
Installation and DeploymentThe compiled binary can now be installed and deployed on the target platform, whether it’s a mobile device, server, embedded system, or another environment.
Execution
ExecutionWhen the program is launched on the target platform, the compiled AOT binary is executed directly by the processor, providing faster startup times and better performance compared to JIT.
Performance and Efficiency
Performance and EfficiencyThe choice of compiler can significantly impact the development and debugging process. Let’s examine the performance and efficiency of AOT and JIT compilers.
JIT Performance
JIT PerformanceThe performance of Just-in-Time (JIT) compilers can vary depending on factors such as the language, the implementation of the JIT compiler, specific optimizations applied, and the characteristics of the code being compiled. Below are some key aspects of JIT compilation performance:
Warm-up Period
Warm-up PeriodJIT compilers typically have a warm-up period during which profiling data is collected, and code is optimized. Initially, the code may run in an interpreted mode or with minimal optimization, leading to reduced performance compared to fully optimized code. However, as more profiling data is gathered and optimizations are applied, the performance improves.
Dynamic Optimization
Dynamic OptimizationJIT compilation allows for dynamic optimization based on runtime profiling. By collecting data about the code’s behavior during execution, JIT can make informed decisions about which optimizations to apply. This flexibility and dynamic behavior provide precise code optimization based on real-time conditions, resulting in highly efficient performance.
Hotspot Optimization
Hotspot OptimizationJIT compilers often focus their optimizations on “hotspots”—sections of the code that are frequently executed. By identifying and optimizing these hotspots, JIT compilers can significantly improve the performance of critical parts of a program.
Multi-Platform Capability
Multi-Platform CapabilityJIT helps achieve compatibility across different platforms. By using an intermediate representation (IR) to abstract platform-specific details, JIT dynamically generates optimized machine code at runtime based on the underlying hardware architecture and operating system. It integrates with portable execution environments or virtual machines (VMs) that manage platform-specific details and leverage platform-specific optimizations when available. JIT can also use cross-compilation techniques to generate code for multiple platforms. These features allow JIT compilers to adapt to various hardware architectures and operating systems, making them versatile and capable of running efficiently across platforms. Developers can write platform-independent code and provide a consistent experience across multiple platforms.
Dynamic Language Features
Dynamic Language FeaturesJIT supports dynamic language features and flexibility, enabling dynamic loading, late binding, and code generation at runtime. These are essential for language features like dynamic typing, reflection, and metaprogramming. JIT provides the ability to inspect and modify code during execution, offering more flexibility and power in programming languages.
Advanced Debugging
Advanced DebuggingJIT compilers offer more advanced debugging capabilities compared to AOT compilers. Since JIT compilers have access to the intermediate representation and perform runtime profiling, they provide precise information about execution, such as function call graphs and memory access patterns. This enables developers to easily identify and track issues in the code with detailed debugging information like line numbers and variable names during runtime. Additionally, JIT compilers often support immediate debugging features, such as attaching a debugger to a running process, inspecting variables, and modifying code behavior during runtime, which is useful for real-time error resolution and bug identification.
Code Sharing and Reusability
Code Sharing and ReusabilityJIT enables code sharing and reusability. By dynamically compiling code, JIT compilers can produce reusable code segments. These segments can be shared across multiple invocations of the same code, reducing redundancy and improving performance. JIT also allows code generation or modification at runtime, enabling dynamic code generation libraries and frameworks to work effectively.
Script Support
Script SupportJIT compilers are widely used in scripting languages because of their ability to execute code without fully compiling the source code and implementing dynamic language features. Scripting languages prioritize rapid development and ease of use, and JIT allows for quick iteration and immediate execution of code changes. With JIT, developers can write and run scripts dynamically and interactively, making it suitable for scenarios like game behavior scripting, web development, or automation tasks.
In general, JIT compilation offers the advantages of dynamic optimization, improved performance, reduced startup times, compatibility across platforms, language flexibility, advanced debugging and profiling capabilities, and code sharing/reusability. These benefits make JIT a valuable approach in many runtime environments and programming language implementations.
AOT Performance
AOT PerformanceThe performance of Ahead-Of-Time (AOT) compilation is generally very good, especially when compared to Just-In-Time (JIT) compilation. Below are the factors that contribute to AOT compilation performance.
Reduced Startup Time
Reduced Startup TimeAOT-compiled code typically has faster startup times compared to JIT-compiled code. This is because the code is compiled into machine code before the program is executed, eliminating the need for a warm-up period or the overhead of runtime compilation. The program can start execution immediately with optimized machine code, resulting in faster startup.
Predictable Performance
Predictable PerformanceAOT provides predictable and stable performance. Since the code is compiled before execution, there are no unexpected pauses or slowdowns caused by dynamic compilation. AOT-compiled code runs at optimized performance right from the start without needing runtime profiling or adaptive optimizations. This predictability is especially useful for real-time systems or applications with strict performance requirements.
Optimized Code
Optimized CodeAOT offers comprehensive optimizations during the compilation process because the compiler has more time and resources to perform thorough analysis and apply complex optimizations. AOT compilers can utilize advanced techniques such as static analysis, interprocedural optimization, and whole-program optimization to produce highly efficient machine code. This results in better performance compared to the limited optimizations available in JIT compilation.
Reduced Memory Usage
Reduced Memory UsageAOT can reduce memory usage compared to JIT compilation. JIT compilers often need to maintain both the intermediate representation (IR) and the compiled machine code simultaneously, which increases memory consumption. AOT-compiled code does not require runtime compilation, and since only the machine code is stored in memory, it reduces memory usage.
Security and Intellectual Property Protection
Security and Intellectual Property ProtectionAOT compilation can enhance security and protect intellectual property. Since the source code is compiled into machine code, it is more difficult to reverse engineer or tamper with compared to interpreted code or code based on an intermediate representation. AOT compilation provides an additional layer of protection against unauthorized access, code modification, or intellectual property theft.
Platform Independence
Platform IndependenceAOT allows for platform independence. The compiled code is specifically generated for the target hardware architecture, enabling the same source code to run on multiple platforms without the need for JIT compilation or platform-specific adaptations. AOT-compiled code can offer portability and consistency across various platforms, making it suitable for hybrid scenarios.
Smaller Output Size
Smaller Output SizeAOT compilation generally results in smaller output sizes compared to JIT compilation. This is due to the elimination of the JIT compiler and its associated runtime infrastructure. AOT allows for comprehensive optimizations during the build process, including techniques such as tree shaking and dead code elimination, reducing the overall code size.
Resource Efficiency
Resource EfficiencyAOT can improve resource efficiency. By performing comprehensive optimizations during the compilation process, AOT compilers can produce code that minimizes energy consumption, reduces CPU usage, and optimizes memory access patterns. This is especially beneficial for resource-constrained environments like mobile devices or embedded systems.
AOT vs. JIT Execution Speed Comparison
AOT vs. JIT Execution Speed ComparisonAs mentioned earlier, AOT-compiled code offers faster application startup times because all the source code is pre-compiled into machine code, making it executable without any delay. Let’s explore and compare some factors affecting execution speed:
JIT-compiled code is slower at startup because it usually requires a warm-up period where the code is initially executed in an interpreted or less-optimized form. Additionally, whenever new code is executed for the first time, the program needs to pause for the JIT compiler to compile it, causing an initial delay. However, once the JIT compiler compiles frequently executed code and identifies hotspots, it applies extensive optimizations, allowing JIT performance to approach AOT levels over time.
AOT compilers have more time and conditions for full optimization during the build phase, allowing for better instruction choices and advanced optimizations, such as inlining, which is challenging for JIT. In contrast, JIT optimizations are restricted to specific code sections within method bodies and have limited time to optimize, whereas AOT can optimize more globally. AOT optimizations occur before execution, while JIT optimizations happen dynamically during runtime.
AOT performs significantly faster for infrequently executed code. While JIT performance may eventually approach AOT for long-running programs, AOT generally retains the advantage.
AOT offers more predictable and stable performance since the code is fully compiled before execution, and no changes occur during runtime. On the other hand, JIT can make real-time decisions based on runtime profiling, allowing the program to adapt to changing conditions, which can be more effective in unpredictable or complex scenarios.
JIT compilers prioritize compilation speed rather than execution speed, producing code quickly. AOT compilers, on the other hand, are specifically optimized for runtime performance.
AOT-compiled code avoids the overhead of a JIT compiler or runtime interpreter, which can improve execution speed. In AOT, only the machine code is needed, whereas JIT requires both compiled code and the source code or intermediate representation to be maintained.
In summary, AOT is faster at startup and for short-lived code, while JIT performance improves over time but usually doesn’t reach the peak performance of AOT in most cases.
Use Cases
Use CasesChoosing the appropriate compiler depends on understanding the specific needs of your application. Factors like performance requirements, the nature of the codebase, expected runtime behavior, and platform limitations should be considered. To make an informed decision between AOT and JIT compilers, it’s essential to consider their use cases.
When to Use JIT?
When to Use JIT?JIT compilers excel when runtime adaptability and flexibility (multi-platform), dynamic optimizations, and advanced debugging are essential. Applications that rely heavily on dynamic language features, reflection, or runtime polymorphism can benefit from JIT compilation.
For example, in web development frameworks like Ruby on Rails or Django, where flexibility and rapid prototyping are crucial, runtime optimizations offered by JIT compilers can improve overall performance.
When to Use AOT?
When to Use AOT?AOT compilers are typically preferred in scenarios with limited hardware resources and real-time requirements. These compilers are commonly used in embedded systems, command-line tools, or applications where fast startup, security, smaller size, resource efficiency, predictable performance, and peak performance are priorities.
For example, in the IoT domain, where low-latency execution and efficient memory usage are critical, AOT compilation can deliver optimized performance even with constrained hardware resources.
Challenges and Limitations
Challenges and LimitationsWhile both compiler approaches offer their own benefits, they also come with specific limitations. In this section, we will explore the limitations and disadvantages of JIT and AOT compilers to help you make a more informed decision.
JIT Limitations
JIT LimitationsThe initial execution of code under JIT compilation can be slower than code compiled by AOT. JIT compilers have a preparation phase during which they collect profiling data and optimize the code. This warm-up period can add overhead and affect the initial performance of the program. Additionally, JIT compilation itself incurs runtime overhead due to the compilation process.
Runtime compilation introduces potential vulnerabilities, such as the risk of code injection attacks.
JIT compilers can negatively impact battery life due to increased CPU usage during code generation and optimization at runtime.
JIT requires additional memory to store the intermediate representation (IR), the compilation cache, and profiling/instrumentation data for performance monitoring.
AOT Limitations
AOT LimitationsCompiling the entire source code into machine code before execution increases build and deployment time, which can slow down the development and iteration process.
AOT compilers generate machine code specifically for the target platform, meaning the code cannot be executed on other platforms without recompilation, limiting cross-platform capability.
AOT applications are highly dependent on the software and hardware environment of the target platform.
AOT compilation lacks the flexibility of JIT. Optimizations performed during AOT are based on pre-execution assumptions and may not be optimized for all runtime scenarios. Changes in execution patterns or environment may require recompilation to take advantage of new optimizations.
Any changes to the code require a complete recompilation and redeployment of the program’s binary.
In Frameworks
In FrameworksIn Java
In JavaBy default, Java code is compiled into bytecode, an intermediate platform-independent representation. The Java Virtual Machine (JVM) then uses a JIT compiler like HotSpot to dynamically compile the bytecode into machine code at runtime. This allows Java applications to benefit from runtime optimizations and cross-platform compatibility. Frameworks like Spring and Hibernate commonly use JIT compilation.
In Flutter
In FlutterFlutter applications are written in the Dart programming language. During development, the Dart Virtual Machine (VM) uses JIT compilation for rapid development and hot-reload functionality. However, when deploying Flutter applications on Android/iOS, the Flutter build process performs AOT compilation of the Dart code, generating optimized machine code for each target platform.
In Rust
In RustRust generally uses AOT compilation instead of JIT. Rust code is compiled directly into machine code using compilers like rustc, rather than being converted into an intermediate representation. Rust emphasizes safety, performance, and control, where AOT excels compared to JIT.
In JavaScript
In JavaScriptJavaScript primarily relies on Just-In-Time (JIT) compilation for execution speed and flexibility, rather than Ahead-Of-Time (AOT) compilation. While WebAssembly provides an option for AOT compilation, the JavaScript engines themselves mostly use JIT.
In .NET Framework and C#
In .NET Framework and C#The .NET Framework uses JIT compilation by default rather than AOT. Code written for .NET (including languages like C#, VB.NET, etc.) is compiled into Microsoft Intermediate Language ( MSIL ), an intermediate representation. At runtime, the Common Language Runtime (CLR) virtual machine converts MSIL into machine code. However, AOT compilation is supported using Ngen.exe, which compiles code before execution.
In Go
In GoGo code is compiled into machine code binaries using Go toolchain compilers such as gc
and gccgo
. Thus, the Go language uses AOT compilation. Go is designed with a focus on features like high performance, stability, and repeatability, which AOT effectively delivers.
The goal of Go is to compile into a binary (machine code) like C/C++ instead of relying on an execution environment like Java/JVM.
Conclusion and Summary
Conclusion and SummaryChoosing between AOT and JIT compilers is not straightforward. Each approach offers distinct advantages and disadvantages, making them more suitable for specific use cases and applications. Developers must carefully assess the needs of their applications and consider factors such as performance, development process, hardware limitations, security, and language compatibility. By selecting the right compiler, developers can optimize their code effectively and maximize the performance of their applications.
Summary of JIT Compilation
Summary of JIT CompilationJIT compilers translate code at runtime and apply dynamic optimizations.
JIT compilers support multi-platform capability by using an intermediate representation independent of the platform.
JIT compilers can accommodate dynamic and flexible scripting and dynamic language features, such as runtime code generation and evaluation.
JIT compilers analyze the runtime behavior of programs and apply optimizations accordingly, which typically results in higher memory usage.
JIT compilers are often more compatible with debuggers, as they generally maintain closer ties with the original source code.
JIT compilers are commonly used in scripting languages, where the ability to execute code on the fly, without prior compilation, is a key feature.
JIT compilers are used in languages such as Java, C#, and JavaScript, offering both performance optimization and flexibility.
Summary of AOT Compilation
Summary of AOT CompilationAOT compilers translate code into machine language before execution and offer more comprehensive optimizations.
AOT-compiled programs run faster since they are pre-compiled into machine code and do not require compilation at runtime.
AOT-compiled code generally consumes less memory compared to JIT-compiled code, as the compact machine code eliminates the need for a JIT compiler during runtime.
AOT compilers optimize code specifically for target hardware architectures and operating systems.
AOT compilers provide greater security, as pre-compiled code has a lower risk of code injection or tampering.
AOT compilers are well-suited for languages with static typing and scenarios where fast startup, memory efficiency, and predictable performance are critical.
AOT compilers are used in languages like C, C++, Rust, and Go, which provide maximum control over system resources and efficient code execution.